As those who know me will know, I am somewhat sceptical when it comes to applying enterprise patterns and practices, such as TDD\unit testing, BDD, DI/IOC etc. to mobile development (mobile apps face a different set of problems to enterprise apps, so why do we feel the need to apply the same solutions?).
Nevertheless unit testing does have it’s place even in mobile apps and TDD is great for solving certain problems. So how do you go about getting started?
Fortunately there are a few projects out there that provide testing frameworks which will allow us to write and run tests on Windows Phone OS. My project of choice is NUnitTestRunner. Unlike other types of projects on WP7 you can’t use an off the shelf test runner like VS2010, ReSharper or TestDriven.NET, since you aren’t going to run your tests on your PC but on your device (or the emulator). Instead you have to create your own test runner by creating your own app to run your tests. Fortunately NUnitTestRunner simplifies all the work involved in creating this app but it does take a little setting up. Heres how:
1.) Download and build the latest release of NUnitTestRunner.
2.) Add a new Windows Phone 7 app to your solution using the “Windows Phone 7 Application” template. Note that we are creating an “Application” here, not a “Class Library” which is where you would normally start with a unit test project (using nUnit, xUnit, MSTest, etc).
3.) Add a reference to the NUnitTestRunnerWP7.dll that was the output of the project you build in step 1.
4.) Remove the NavigationPage attribute from the <Tasks> element in the WMAppManifest.xml.

5.) Delete the MainPage.xaml file from your project since it is no longer required.
6.) Remove the <Application.ApplicationLifeTimeObjects> elements from App.xaml.

7.) Replace all the code in App.xaml.cs with the following:
public partial class App : Application
{
public App()
{
InitializeComponent();
this.Startup += App_Startup;
}
void App_Startup(object sender, StartupEventArgs e)
{
var assembliesList = new[]
{
//This assumes all tests are in this
// assembly. Add other assemblies
// to the array if not.
Assembly.GetExecutingAssembly()
};
NUnitTestRunnerWP7.NUnitTestRunner.Setup(assembliesList);
}
}
Now set Visual Studio to run this test app on startup – right click on the project in Solution Explorer and choose “Set as Startup Project”. Now when you press F5 to debug it should launch the test runner. You should see this:
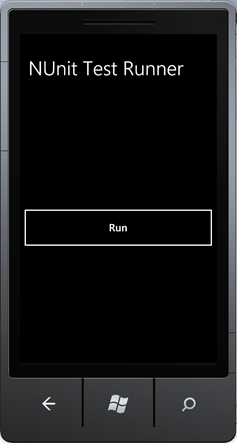
If you click the Run button you should see this:

We see 0 Passed and 0 Failed but we haven’t written any tests so that is to be expected.
8.) Finally start adding some test fixtures just as you usually do in NUnit.
[TestFixture]
public class ExternalSampleTests
{
[Test]
public void ExternalSampleTest()
{
Assert.IsTrue(true);
}
}
Running your project with some test in it should yield something along the lines of:
